Cooperative Interruption of a Thread in C++20: Callbacks
I introduced in my last post “Cooperative Interruption of a Thread in C++20” callbacks. Today, I dive deeper.
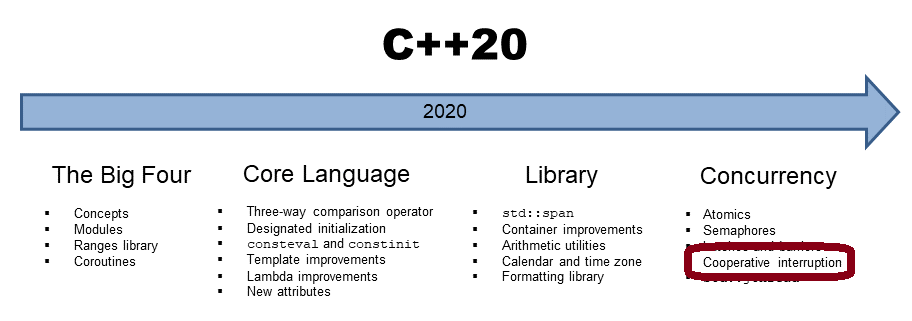
First , here’s a short reminder.
Reminder
In my last post “Cooperative Interruption of a Thread in C++20“, I presented the following program.
// invokeCallback.cpp #include <chrono> #include <iostream> #include <thread> #include <vector> using namespace::std::literals; auto func = [](std::stop_token stoken) { // (1) int counter{0}; auto thread_id = std::this_thread::get_id(); std::stop_callback callBack(stoken, [&counter, thread_id] { // (2) std::cout << "Thread id: " << thread_id << "; counter: " << counter << '\n'; }); while (counter < 10) { std::this_thread::sleep_for(0.2s); ++counter; } }; int main() { std::cout << '\n'; std::vector<std::jthread> vecThreads(10); for(auto& thr: vecThreads) thr = std::jthread(func); std::this_thread::sleep_for(1s); // (3) for(auto& thr: vecThreads) thr.request_stop(); // (4) std::cout << '\n'; }
Each of the ten threads invokes the lambda function func
(1). The callback (2) displays the thread id
and the counter
. Due to the one-second sleeping of the main thread (3) and the sleeping of the child threads, the counter is 4 when the callbacks are invoked. The call thr.request_stop()
triggers the callback on each thread.
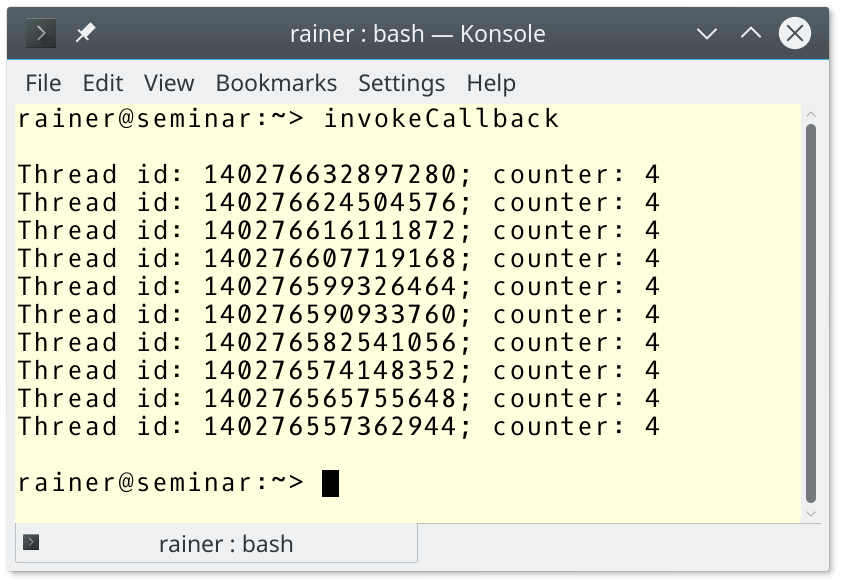
One question was not answered in my last post:
Where does the callback run?
The std::stop_callback
constructor registers the callback function for the std::stop_token
given by the associated std::stop_source.
This callback function is either invoked in the thread invoking request_stop()
or the thread constructing the std::stop_callback.
If the request to stop happens prior to the registration of the std::stop_callback
, the callback is invoked in the thread constructing the std::stop_callback.
Otherwise, the callback is invoked in the thread invoking request_stop.
If the call request_stop()
happens after the execution of the thread constructing the std::stop_callback
, the registered callback will never be called.
You can register more than one callback for one or more threads using the same std::stop_token.
The C++ standard does not guarantee the order in which they are executed.
More than one callback
// invokeCallbacks.cpp #include <chrono> #include <iostream> #include <thread> using namespace std::literals; void func(std::stop_token stopToken) { std::this_thread::sleep_for(100ms); for (int i = 0; i <= 9; ++i) { std::stop_callback cb(stopToken, [i] { std::cout << i; }); } std::cout << '\n'; } int main() { std::cout << '\n'; std::jthread thr1 = std::jthread(func); std::jthread thr2 = std::jthread(func); thr1.request_stop(); thr2.request_stop(); std::cout << '\n'; }
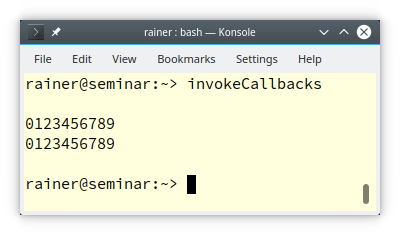
A General Mechanism to Send Signals
The pair std::stop_source
and std::stop_token
can be considered as a general mechanism to send a signal. By copying the std::stop_token
, you can send the signal to any entity executing something. In the following example, I use std::async,
std::promise, std::thread
, and std::jthread
in various combinations.
// signalStopRequests.cpp #include <iostream> #include <thread> #include <future> using namespace std::literals; void function1(std::stop_token stopToken, const std::string& str){ std::this_thread::sleep_for(1s); if (stopToken.stop_requested()) std::cout << str << ": Stop requested\n"; } void function2(std::promise<void> prom, std::stop_token stopToken, const std::string& str) { std::this_thread::sleep_for(1s); std::stop_callback callBack(stopToken, [&str] { std::cout << str << ": Stop requested\n"; }); prom.set_value(); } int main() { std::cout << '\n'; std::stop_source stopSource; // (1) std::stop_token stopToken = std::stop_token(stopSource.get_token()); // (2) std::thread thr1 = std::thread(function1, stopToken, "std::thread"); // (3) std::jthread jthr = std::jthread(function1, stopToken, "std::jthread"); // (4) auto fut1 = std::async([stopToken] { // (5) std::this_thread::sleep_for(1s); if (stopToken.stop_requested()) std::cout << "std::async: Stop requested\n"; }); std::promise<void> prom; // (6) auto fut2 = prom.get_future(); std::thread thr2(function2, std::move(prom), stopToken, "std::promise"); stopSource.request_stop(); // (7) if (stopToken.stop_requested()) std::cout << "main: Stop requested\n"; // (8) thr1.join(); thr2.join(); std::cout << '\n'; }
Thanks to the stopSource
(line 1), I can create the stopToken
(line 2) for each running entity, such as std::thread
(line 3), std::jthread
(line 4), std::async
(line 5), or std::promise
(line 6). A std::stop_token
is cheap to copy. Line 7 triggers stopSource.request_stop.
Also, the main thread (line 8) gets the signal. I use in this example std::jthread. std::jthread
, and std::condition_variable_any
has explicit member functions to deal with cooperative interruption more conveniently. Read more about it in the following post: “An Improved Thread with C++20“.
What’s next?
I will take a break in the next two weeks. Afterward, I will jump again in C++23 and add the first time in C++26.
Modernes C++ Mentoring
Do you want to stay informed: Subscribe.
Thanks a lot to my Patreon Supporters: Matt Braun, Roman Postanciuc, Tobias Zindl, G Prvulovic, Reinhold Dröge, Abernitzke, Frank Grimm, Sakib, Broeserl, António Pina, Sergey Agafyin, Андрей Бурмистров, Jake, GS, Lawton Shoemake, Jozo Leko, John Breland, Venkat Nandam, Jose Francisco, Douglas Tinkham, Kuchlong Kuchlong, Robert Blanch, Truels Wissneth, Mario Luoni, Friedrich Huber, lennonli, Pramod Tikare Muralidhara, Peter Ware, Daniel Hufschläger, Alessandro Pezzato, Bob Perry, Satish Vangipuram, Andi Ireland, Richard Ohnemus, Michael Dunsky, Leo Goodstadt, John Wiederhirn, Yacob Cohen-Arazi, Florian Tischler, Robin Furness, Michael Young, Holger Detering, Bernd Mühlhaus, Stephen Kelley, Kyle Dean, Tusar Palauri, Juan Dent, George Liao, Daniel Ceperley, Jon T Hess, Stephen Totten, Wolfgang Fütterer, Matthias Grün, Phillip Diekmann, Ben Atakora, Ann Shatoff, Rob North, Bhavith C Achar, Marco Parri Empoli, Philipp Lenk, Charles-Jianye Chen, Keith Jeffery, Matt Godbolt, Honey Sukesan, bruce_lee_wayne, Silviu Ardelean, and Seeker.
Thanks, in particular, to Jon Hess, Lakshman, Christian Wittenhorst, Sherhy Pyton, Dendi Suhubdy, Sudhakar Belagurusamy, Richard Sargeant, Rusty Fleming, John Nebel, Mipko, Alicja Kaminska, Slavko Radman, and David Poole.
My special thanks to Embarcadero | ![]() |
My special thanks to PVS-Studio | ![]() |
My special thanks to Tipi.build | ![]() |
My special thanks to Take Up Code | ![]() |
My special thanks to SHAVEDYAKS | ![]() |
Modernes C++ GmbH
Modernes C++ Mentoring (English)
Rainer Grimm
Yalovastraße 20
72108 Rottenburg
Mail: schulung@ModernesCpp.de
Mentoring: www.ModernesCpp.org