Constant Expressions with constexpr
You can define with the keyword constexpr an expression that can be evaluated at compile time. constexpr can be used for variables, functions, and user-defined types. An expression that is evaluated at compile time has a lot of advantages. For example, constexpr variables and instances of user-defined types are automatically thread-safe and can be stored in ROM; constexpr functions evaluated at compile-time are done with their work at run time.
All done at compile time
I already have indicated it in my post on User-defined literals. The calculation of how many kilometers per week I drive on average has a massive optimization potential. In this post, I will solve my promise. To make it easy for you. Here is the program from the post User-defined literals once more.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 |
// userdefinedLiterals.cpp #include <iostream> namespace Distance{ class MyDistance{ public: MyDistance(double i):m(i){} friend MyDistance operator+(const MyDistance& a, const MyDistance& b){ return MyDistance(a.m + b.m); } friend MyDistance operator-(const MyDistance& a,const MyDistance& b){ return MyDistance(a.m - b.m); } friend MyDistance operator*(double m, const MyDistance& a){ return MyDistance(m*a.m); |
How can I massively improve the program? Relatively easy by using constexpr. My key idea is to declare all instances of MyDistance in the main program as constexpr. Therefore, I say to the compiler: Instantiate the objects at compile time. But the compiler can only perform its job if the instantiation is based on constant expressions. I will get a compiler error if the compiler can not do it.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 |
// userdefinedLiteralsConstexpr.cpp #include <iostream> namespace Distance{ class MyDistance{ public: constexpr MyDistance(double i):m(i){} friend constexpr MyDistance operator+(const MyDistance& a, const MyDistance& b){ return MyDistance(a.m + b.m); } friend constexpr MyDistance operator-(const MyDistance& a,const MyDistance& b){ return MyDistance(a.m - b.m); } friend constexpr MyDistance operator*(double m, const MyDistance& a){ return MyDistance(m*a.m); } friend constexpr MyDistance operator/(const MyDistance& a, int n){ return MyDistance(a.m/n); } friend std::ostream& operator<< (std::ostream &out, const MyDistance& myDist){ out << myDist.m << " m"; return out; } private: |
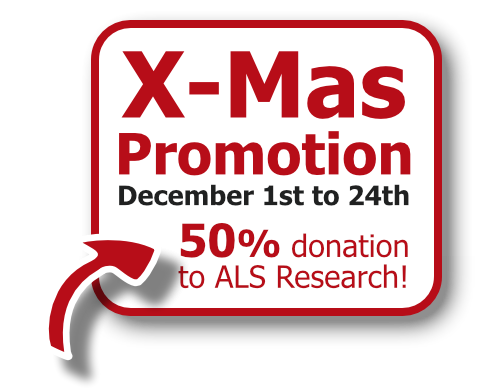
Modernes C++ Mentoring
Do you want to stay informed: Subscribe.
The result of the calculation is not so exciting. To compile the program, I have to use a C++14 compiler. A current GCC of clang is fine. The current Microsoft Visual 2015 C++-Compiler supports only C++11. Therefore, cl.exe will not compile the function getAverageDistance. In C++11, a constexpr function can only have a return statement.
But it is very exciting to look at the assembler instructions. To simplify my job, I use the interactive compiler hosted on https://gcc.godbolt.org/. Have I already mentioned that I like this tool very much?
How should we interpret the results? Quite easy. In the main program (lines 64 – 75) defined constant expressions are part of the assembler program. Or to say it differently. All calculations are done at compile time. At run time, the program consists only of the already calculated expressions. That is an extremely easy job for the run time.
What’s next?
So, that’s enough to make you keen on more. The details will follow in the next post. I will look deeper into constexpr variables, functions, and user-defined types. You have to keep a few rules in mind. Firstly, C++14 constexpr functions are more powerful than C++11 constexpr functions. Secondly, you can execute constexpr functions at run time. Lastly, there are a few restrictions for user-defined types to instantiate them at compile time.
Thanks a lot to my Patreon Supporters: Matt Braun, Roman Postanciuc, Tobias Zindl, G Prvulovic, Reinhold Dröge, Abernitzke, Frank Grimm, Sakib, Broeserl, António Pina, Sergey Agafyin, Андрей Бурмистров, Jake, GS, Lawton Shoemake, Jozo Leko, John Breland, Venkat Nandam, Jose Francisco, Douglas Tinkham, Kuchlong Kuchlong, Robert Blanch, Truels Wissneth, Mario Luoni, Friedrich Huber, lennonli, Pramod Tikare Muralidhara, Peter Ware, Daniel Hufschläger, Alessandro Pezzato, Bob Perry, Satish Vangipuram, Andi Ireland, Richard Ohnemus, Michael Dunsky, Leo Goodstadt, John Wiederhirn, Yacob Cohen-Arazi, Florian Tischler, Robin Furness, Michael Young, Holger Detering, Bernd Mühlhaus, Stephen Kelley, Kyle Dean, Tusar Palauri, Juan Dent, George Liao, Daniel Ceperley, Jon T Hess, Stephen Totten, Wolfgang Fütterer, Matthias Grün, Phillip Diekmann, Ben Atakora, Ann Shatoff, Rob North, Bhavith C Achar, Marco Parri Empoli, Philipp Lenk, Charles-Jianye Chen, Keith Jeffery, Matt Godbolt, and Honey Sukesan.
Thanks, in particular, to Jon Hess, Lakshman, Christian Wittenhorst, Sherhy Pyton, Dendi Suhubdy, Sudhakar Belagurusamy, Richard Sargeant, Rusty Fleming, John Nebel, Mipko, Alicja Kaminska, Slavko Radman, and David Poole.
My special thanks to Embarcadero | ![]() |
My special thanks to PVS-Studio | ![]() |
My special thanks to Tipi.build | ![]() |
My special thanks to Take Up Code | ![]() |
My special thanks to SHAVEDYAKS | ![]() |
Modernes C++ GmbH
Modernes C++ Mentoring (English)
Rainer Grimm
Yalovastraße 20
72108 Rottenburg
Mail: schulung@ModernesCpp.de
Mentoring: www.ModernesCpp.org
Modernes C++ Mentoring,
Leave a Reply
Want to join the discussion?Feel free to contribute!