An Overview of C++26: Core Language
C++26 has a lot to offer. Let me directly jump in and give you an overview.
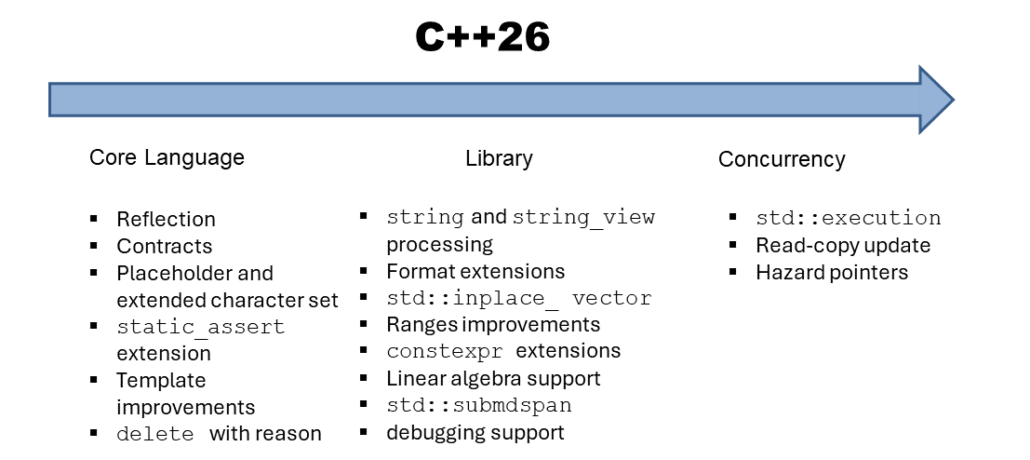
This image gives you the first idea of what’s inside or outside C++26. Writing a blog post about the future is always challenging because the design freeze of C++26 is in the first quarter of 2025.
Fishing in Murky Waters
This image should only give you the first idea of what’s inside or outside C++26. I will adapt the image while writing the next months about C++26 if necessary. For example, the three powerful features reflection, contracts, and std::execution
made a big step towards their standardization. On the contrary, I intentionally ignore pattern matching on the image.
Very impactful features such as reflection or contracts implement the agile idea of the minimum viable product. “A minimum viable product (MVP) is a version of a product with just enough features to be usable by early customers who can then provide feedback for future product development.” (https://en.wikipedia.org/wiki/Minimum_viable_product). This means C++26 is only the starting point for features such as reflection or contracts.
If possible, I show you the feature in action. Many features are already implemented in the brand-new C++ compilers. For the rest, I hope for prototype implementations.
Let me start with the core language.
Core Language
Reflection
Reflection is the ability of a program to examine, introspect, and modify its structure and behavior. This makes compile-time programming in C++ much more powerful.
I don’t want to annoy you with too much theory in this overview post. Therefore, I will show you my favorite example from the reflection proposal P2996R5.
A question I often have to answer in my classes is: How could I convert an enumerator to a string?
// enumString.cpp
#include <iostream>
#include <experimental/meta>
#include <string>
#include <type_traits>
// start 'expand' definition
namespace __impl {
template<auto... vals>
struct replicator_type {
template<typename F>
constexpr void operator>>(F body) const {
(body.template operator()<vals>(), ...);
}
};
template<auto... vals>
replicator_type<vals...> replicator = {};
}
template<typename R>
consteval auto expand(R range) {
std::vector<std::meta::info> args;
for (auto r : range) {
args.push_back(std::meta::reflect_value(r));
}
return substitute(^__impl::replicator, args);
}
// end 'expand' definition
template<typename E>
requires std::is_enum_v<E> // (1)
constexpr std::string enum_to_string(E value) {
std::string result = "<unnamed>";
[:expand(std::meta::enumerators_of(^E)):] >> // (2)
[&]<auto e>{
if (value == [:e:]) {
result = std::meta::identifier_of(e); // (3)
}
};
return result;
}
int main() {
std::cout << '\n';
enum Color { red, green, blue };
std::cout << "enum_to_string(Color::red): " << enum_to_string(Color::red) << '\n';
// std::cout << "enum_to_string(42): " << enum_to_string(42) << '\n';
std::cout << '\n';
}
This example uses the experimental features (std::meta
) of the standard.
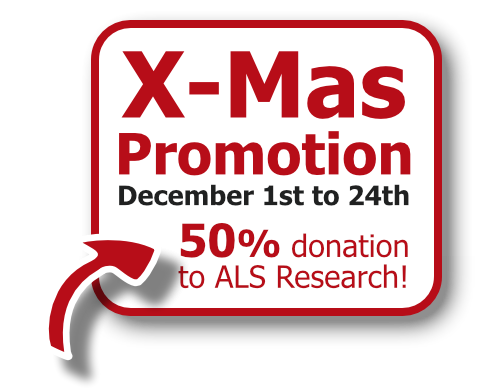
Modernes C++ Mentoring
Do you want to stay informed: Subscribe.
Here’s the output of the program:

Let me say a few words about the function, template enum_to_string
. The function expand
is a workaround. The function call enum_to_string(Color(42))
breaks because the function requires an enum.: requires std::is_enum_v<E>
(line 1).
Line (1) applies a reflection operator (^E
) and calls the meta function enum_to_std::meta::enumerators_of(^E))
. Finally, the so called splicer ([: ref
l :]
) produces in line (2) grammatical elements for reflection. The second meta function in line (3) creates the string:std:meta::identifier_of(e))
. The consteval
meta functions are executed at compile time, and so is the reflection.
Contracts
A contract specifies interfaces for software components precisely and checkably. These software components are functions that fulfill preconditions, postconditions, and invariants.
Here’s a straightforward example from the proposal P2900.
int f(const int x)
pre (x != 1) // a precondition assertion
post(r : r != 2) // a postcondition assertion; r refers to the return value of f
{
contract_assert (x != 3); // an assertion statement
return x;
}
The function f has a precondition, postcondition, and invariant. The precondition is checked before the function Invocation, the postcondition after the function invocation, and the invariant precisely at the point of its invocation.
Invoking the function with arguments 1, 2, or 3 causes a contract violation. There are different ways to react to a contract violation.
void g()
{
f(0); // no contract violation
f(1); // violates precondition assertion of f
f(2); // violates postcondition assertion of f
f(3); // violates assertion statement within f
f(4); // no contract violation
}
The core language of C++26 has more to offer besides reflection and contracts. Let me name them and present a code snippet from the corresponding proposals.
- Placeholder and extended character set
auto [x, y, _] = f();
The _ stands for I don’t care and it can be used more than once.
static_assert
extension
static_assert(sizeof(S) == 1, std::format("Unexpected sizeof: expected 1, got {}", sizeof(S))
- Template improvements
There are many template improvements in C++26. My favorite one is pack indexing:
template <typename... T> constexpr auto first_plus_last(T... values) -> T...[0] { return T...[0](values...[0] + values...[sizeof...(values)-1]); } int main() { //first_plus_last(); // ill formed static_assert(first_plus_last(1, 2, 10) == 11); }
delete
with reason
delete("Should have a reason");
What’s Next?
In my next post, I will overview the C++26 library.
Thanks a lot to my Patreon Supporters: Matt Braun, Roman Postanciuc, Tobias Zindl, G Prvulovic, Reinhold Dröge, Abernitzke, Frank Grimm, Sakib, Broeserl, António Pina, Sergey Agafyin, Андрей Бурмистров, Jake, GS, Lawton Shoemake, Jozo Leko, John Breland, Venkat Nandam, Jose Francisco, Douglas Tinkham, Kuchlong Kuchlong, Robert Blanch, Truels Wissneth, Mario Luoni, Friedrich Huber, lennonli, Pramod Tikare Muralidhara, Peter Ware, Daniel Hufschläger, Alessandro Pezzato, Bob Perry, Satish Vangipuram, Andi Ireland, Richard Ohnemus, Michael Dunsky, Leo Goodstadt, John Wiederhirn, Yacob Cohen-Arazi, Florian Tischler, Robin Furness, Michael Young, Holger Detering, Bernd Mühlhaus, Stephen Kelley, Kyle Dean, Tusar Palauri, Juan Dent, George Liao, Daniel Ceperley, Jon T Hess, Stephen Totten, Wolfgang Fütterer, Matthias Grün, Phillip Diekmann, Ben Atakora, Ann Shatoff, Rob North, Bhavith C Achar, Marco Parri Empoli, Philipp Lenk, Charles-Jianye Chen, Keith Jeffery, Matt Godbolt, and Honey Sukesan.
Thanks, in particular, to Jon Hess, Lakshman, Christian Wittenhorst, Sherhy Pyton, Dendi Suhubdy, Sudhakar Belagurusamy, Richard Sargeant, Rusty Fleming, John Nebel, Mipko, Alicja Kaminska, Slavko Radman, and David Poole.
My special thanks to Embarcadero | ![]() |
My special thanks to PVS-Studio | ![]() |
My special thanks to Tipi.build | ![]() |
My special thanks to Take Up Code | ![]() |
My special thanks to SHAVEDYAKS | ![]() |
Modernes C++ GmbH
Modernes C++ Mentoring (English)
Rainer Grimm
Yalovastraße 20
72108 Rottenburg
Mail: schulung@ModernesCpp.de
Mentoring: www.ModernesCpp.org
Modernes C++ Mentoring,