C++26 Library: string and string_view Processing
C++26 offers many small improvements around string
s and string_view
s.
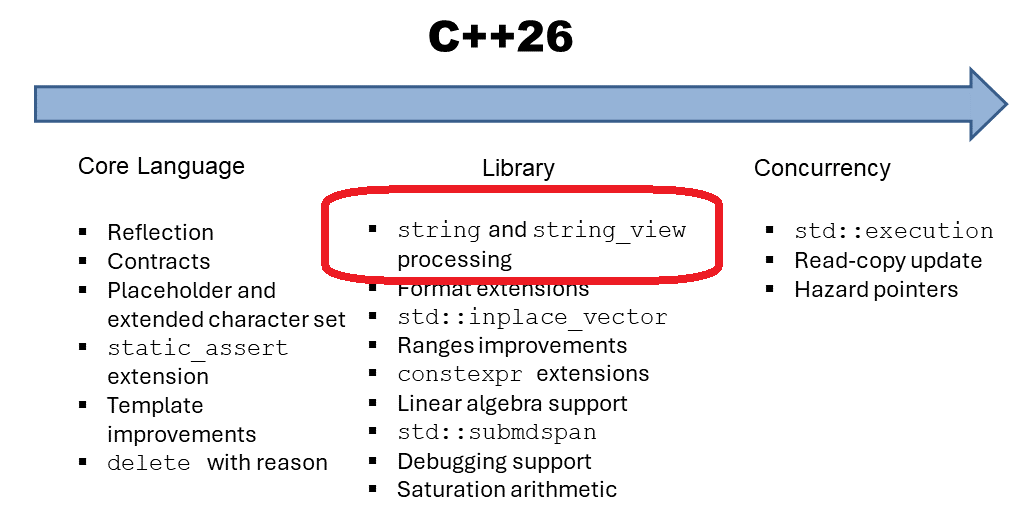
First of all: What is a string_view?
std::string_view
A std::string_view
is a non-owning reference to a string
. It represents a view of a sequence of characters. This sequence of characters can be a C++ string or a C-string. In a typical way, C++17 offers four type synonyms for the underlying character types.
std::string_view std::basic_string_view<char> std::wstring_view std::basic_string_view<wchar_t> std::u16string_view std::basic_string_view<char16_t> std::u32string_view std::basic_string_view<char32_t>
One question remains. Why do we need a std::string_view
? Why had Google, LLVM, and Bloomberg already an implementation of a string view? The answer is easy. It’s pretty cheap to copy a std::string_view.
A std::string_view
only needs two pieces of information: the pointer to the character sequence and their length. As you may assume, the std::string_view
and its three siblings consist mainly of reading operations that follow the interface of std::string.
Mainly because it gets the new methods remove_prefix
and remove_suffix
.
Testing for Success or Failure of <charconv
> Functions
The functions std::to_chars
and std::from_chars
was inconvenient to test: if(res.ec == std::errc{})
.
Here’s a simplified program from https://en.cppreference.com/w/cpp/utility/to_chars.
// charconv.cpp #include <charconv> #include <iomanip> #include <iostream> #include <string_view> #include <system_error> template <typename T> void show_to_chars(T value) { const size_t buf_size = 5; char buf[buf_size]; std::to_chars_result result = std::to_chars(buf, buf + buf_size, value); if (result.ec != std::errc{}) std::cout << std::make_error_code(result.ec).message() << '\n'; else{ std::string_view str(buf, result.ptr - buf); std::cout << std::quoted(str) << '\n'; } } int main() { show_to_chars(42); show_to_chars(1234567); }
The program includes the headers <charconv>
for character conversion, <iomanip>
for input/output manipulators, <string_view>
for handling string_view
s, and <system_error>
for error handling.
The show_to_chars
function template takes a value of any type T
and tries to convert it to a character sequence. Inside the function, a buffer of size 5 is declared to hold the resulting character sequence. The std::to_chars
function is then called to perform the conversion, storing the result in a std::to_chars_result
object named result
.
The result
object contains a pointer to the end of the converted character sequence and an error code. If the error code is not equal to std::errc{}
, indicating an error occurred during conversion, an error message is printed to the console using std::make_error_code(result.ec).message()
. Otherwise, a std::string_view
object is created to represent the converted character sequence, and the sequence is printed to the console using std::quoted
to ensure it is displayed within double quotes.
In the main
function, the show_to_chars
function is called twice: first with the value 42
and then with the value 1234567
. The first call successfully converts the value 42
to a character sequence and prints it. However, the second call attempts to convert the value 1234567
, which exceeds the buffer size of 5, resulting in an error message being printed to the console.
Modernes C++ Mentoring
Do you want to stay informed: Subscribe.
Finally, here comes the output of the program:

Thanks to C++26, the function std::to_chars
retuns a boolean and the function show_to_chars
can be simplified:
template <typename T> void show_to_chars(T value) { std::array<char, 5> str; if (auto result = std::to_chars(str.data(), str.data() + str.size(), value)) { std::string_view strView(str.data(), result.ptr); std::cout << std::quoted(strView) << '\n'; } else std::cout << std::make_error_code(result.ec).message() << '\n'; }
Inside the function, a std::array
of characters with a fixed size of 5 is declared to hold the resulting character sequence. The std::to_chars
function is then called to perform the conversion. This function attempts to convert the numeric value value
into a character sequence and store it in the str
array. The std::to_chars
function returns a std::to_chars_result
object, which contains a pointer to the end of the converted character sequence and an error code.
The function uses an if
statement to check the result of the std::to_chars
call. If the conversion is successful, the result
object will be implicitly convertible to true
, and the function will proceed to print the converted character sequence. This is done by creating a std::string_view
object named strView
that represents the character sequence from the beginning of the str
array up to the result.ptr
pointer. The std::cout
stream is then used to print this string view to the console, with std::quoted
ensuring the output is displayed within double quotes.
If the conversion fails, the result
bject will be implicitly convertible to false
, and the function will print an error message instead. The error message is obtained by calling std::make_error_code(result.ec).message()
, which converts the error code to a human-readable string.
They are further string
and string_view
processing functions in C++26:
- Interfacing
stringstream
s withstd::string_view
- Concatenation of
string
s andstring_view
s - Arithmetic overloads of
std::to_string
and usestd::format
I already presented them in my previous post: “An Overview of C++26: The Library“.
What’s Next?
The C++26 library has more to offer.
Thanks a lot to my Patreon Supporters: Matt Braun, Roman Postanciuc, Tobias Zindl, G Prvulovic, Reinhold Dröge, Abernitzke, Frank Grimm, Sakib, Broeserl, António Pina, Sergey Agafyin, Андрей Бурмистров, Jake, GS, Lawton Shoemake, Jozo Leko, John Breland, Venkat Nandam, Jose Francisco, Douglas Tinkham, Kuchlong Kuchlong, Robert Blanch, Truels Wissneth, Mario Luoni, Friedrich Huber, lennonli, Pramod Tikare Muralidhara, Peter Ware, Daniel Hufschläger, Alessandro Pezzato, Bob Perry, Satish Vangipuram, Andi Ireland, Richard Ohnemus, Michael Dunsky, Leo Goodstadt, John Wiederhirn, Yacob Cohen-Arazi, Florian Tischler, Robin Furness, Michael Young, Holger Detering, Bernd Mühlhaus, Stephen Kelley, Kyle Dean, Tusar Palauri, Juan Dent, George Liao, Daniel Ceperley, Jon T Hess, Stephen Totten, Wolfgang Fütterer, Matthias Grün, Phillip Diekmann, Ben Atakora, Ann Shatoff, Rob North, Bhavith C Achar, Marco Parri Empoli, Philipp Lenk, Charles-Jianye Chen, Keith Jeffery, Matt Godbolt, and Honey Sukesan.
Thanks, in particular, to Jon Hess, Lakshman, Christian Wittenhorst, Sherhy Pyton, Dendi Suhubdy, Sudhakar Belagurusamy, Richard Sargeant, Rusty Fleming, John Nebel, Mipko, Alicja Kaminska, Slavko Radman, and David Poole.
My special thanks to Embarcadero | ![]() |
My special thanks to PVS-Studio | ![]() |
My special thanks to Tipi.build | ![]() |
My special thanks to Take Up Code | ![]() |
My special thanks to SHAVEDYAKS | ![]() |
Modernes C++ GmbH
Modernes C++ Mentoring (English)
Rainer Grimm
Yalovastraße 20
72108 Rottenburg
Mail: schulung@ModernesCpp.de
Mentoring: www.ModernesCpp.org
Modernes C++ Mentoring,