Improved Iterators with Ranges
There are more reasons to prefer ranges library above the classical Standard Template Library. The ranges iterators support unified lookup rules and provide additional safety guarantees.
Unified Lookup Rules
Assume you want to implement a generic function that calls begin
on a given container. The question is if the function calling begin
on a container should assume a free begin
function or a member function begin
?
// begin.cpp #include <cstddef> #include <iostream> #include <ranges> struct ContainerFree { // (1) ContainerFree(std::size_t len): len_(len), data_(new int[len]){} size_t len_; int* data_; }; int* begin(const ContainerFree& conFree) { // (2) return conFree.data_; } struct ContainerMember { // (3) ContainerMember(std::size_t len): len_(len), data_(new int[len]){} int* begin() const { // (4) return data_; } size_t len_; int* data_; }; void callBeginFree(const auto& cont) { // (5) begin(cont); } void callBeginMember(const auto& cont) { // (6) cont.begin(); } int main() { const ContainerFree contFree(2020); const ContainerMember contMemb(2023); callBeginFree(contFree); callBeginMember(contMemb); callBeginFree(contMemb); // (7) callBeginMember(contFree); // (8) }
ContainerFree
(line 1) has a free function begin
(line 2), and ContainerMember
(line 3) has a member function begin
(line 4). Accordingly, contFree
can use the generic function callBeginFree
using the free function call begin(cont)
(line 5), and contMemb
can use the generic function callBeginMember
using the member function call cont.begin
(line 6). When I invoke callBeginFree
and callBeginMember
The compilation fails with the inappropriate containers in lines (7) and (8).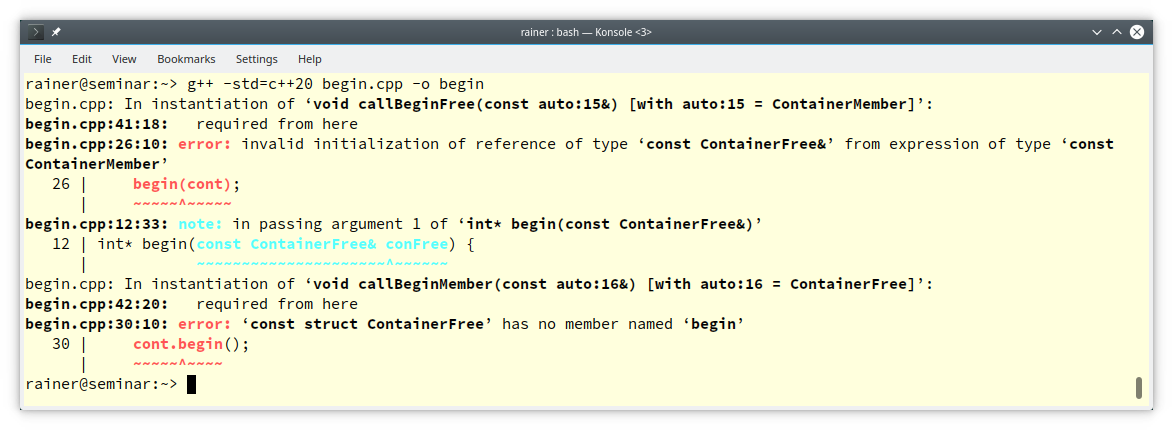
I can solve this issue by providing two different begin
implementations: classical and range based.
// beginSolved.cpp #include <cstddef> #include <iostream> #include <ranges> struct ContainerFree { ContainerFree(std::size_t len): len_(len), data_(new int[len]){} size_t len_; int* data_; }; int* begin(const ContainerFree& conFree) { return conFree.data_; } struct ContainerMember { ContainerMember(std::size_t len): len_(len), data_(new int[len]){} int* begin() const { return data_; } size_t len_; int* data_; }; void callBeginClassical(const auto& cont) { using std::begin; // (1) begin(cont); } void callBeginRanges(const auto& cont) { std::ranges::begin(cont); // (2) } int main() { const ContainerFree contFree(2020); const ContainerMember contMemb(2023); callBeginClassical(contFree); callBeginRanges(contMemb); callBeginClassical(contMemb); callBeginRanges(contFree); }
The classical way to solve this issue is to bring std::begin
into the scope with a so-called using-declaration (line 1). Thanks to ranges, you can directly use std::ranges::begin
(line 2). std::ranges::begin
considers both implementations of begin
: the free version and the member function.
Finally, let me write about safety.
Safety
Let me start with iterators.
Modernes C++ Mentoring
Do you want to stay informed: Subscribe.
Iterators
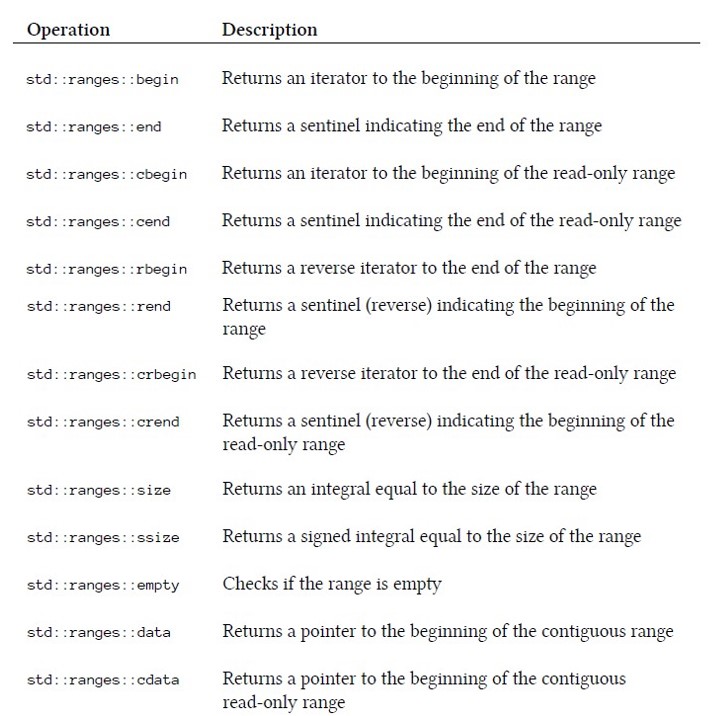
There is a big difference when you use these operations for accessing the underlying range. The compilation fails when you use the range access on the std::ranges
‘s variant if the argument is an rvalue. On the contrary, using the same operation from the classical std
namespace is undefined behavior.
// rangesAccess.cpp #include <iterator> #include <ranges> #include <vector> int main() { auto beginIt1 = std::begin(std::vector<int>{1, 2, 3}); auto beginIt2 = std::ranges::begin(std::vector<int>{1, 2, 3}); }
std::ranges::begin
provides only overloads for lvalues. The temporary vector std::vector{1, 2, 3}
is an rvalue. Consequentially, the compilation of the program fails.
The abbreviations lvalue and rvalue stand for locatable value and readable value.
- lvalue (locatable value): A locatable value is an object with a location in memory, and you can, therefore, determine its address. An lvalue has an identity.
- rvalue (readable value): A rvalue is a value you can only read from. It does not represent an object in memory, and you cannot determine its address.
I must admit that my short explanations of lvalues and rvalues are a simplification. If you want more details about value categories, read the following post, Value Categories.
By the way, iterators and views provide these additional safety guarantees.
Views
Views do not own data. Therefore, views do not extend the lifetime of their data. Consequently, views can only be created on lvalues. The compilation fails if you create a view on a temporary range.
// temporaryRange.cpp #include <initializer_list> #include <ranges> int main() { const auto numbers = {1, 2, 3, 4, 5}; auto firstThree = numbers | std::views::drop(3); // (1) // auto firstThree = {1, 2, 3, 4, 5} | std::views::drop(3); // (2) std::ranges::drop_view firstFour{numbers, 4}; // (3) // std::ranges::drop_view firstFour{{1, 2, 3, 4, 5}, 4}; // (4) }
All is fine when lines 1 and 3 are used with the lvalue numbers. On the contrary, using the commented-out lines 2 and 4 on the rvalue std::initializer_list<int> {1, 2, 3, 4, 5}
, causes the GCC compiler to complain verbosely:
What’s next?
In my next post, I will first peek into the C++23 future. In particular, the ranges library will get many improvements. There is with std::ranges::to
a convenient way to construct containers from ranges. Additionally, we will get almost twenty new algorithms. Here are a few of them: std::views::chunk_by, std::views::slide, std::views::join_with, std::views::zip_transform,
and std::views::adjacent_transform
.
Thanks a lot to my Patreon Supporters: Matt Braun, Roman Postanciuc, Tobias Zindl, G Prvulovic, Reinhold Dröge, Abernitzke, Frank Grimm, Sakib, Broeserl, António Pina, Sergey Agafyin, Андрей Бурмистров, Jake, GS, Lawton Shoemake, Jozo Leko, John Breland, Venkat Nandam, Jose Francisco, Douglas Tinkham, Kuchlong Kuchlong, Robert Blanch, Truels Wissneth, Mario Luoni, Friedrich Huber, lennonli, Pramod Tikare Muralidhara, Peter Ware, Daniel Hufschläger, Alessandro Pezzato, Bob Perry, Satish Vangipuram, Andi Ireland, Richard Ohnemus, Michael Dunsky, Leo Goodstadt, John Wiederhirn, Yacob Cohen-Arazi, Florian Tischler, Robin Furness, Michael Young, Holger Detering, Bernd Mühlhaus, Stephen Kelley, Kyle Dean, Tusar Palauri, Juan Dent, George Liao, Daniel Ceperley, Jon T Hess, Stephen Totten, Wolfgang Fütterer, Matthias Grün, Phillip Diekmann, Ben Atakora, Ann Shatoff, Rob North, Bhavith C Achar, Marco Parri Empoli, Philipp Lenk, Charles-Jianye Chen, Keith Jeffery, Matt Godbolt, Honey Sukesan, bruce_lee_wayne, Silviu Ardelean, and Seeker.
Thanks, in particular, to Jon Hess, Lakshman, Christian Wittenhorst, Sherhy Pyton, Dendi Suhubdy, Sudhakar Belagurusamy, Richard Sargeant, Rusty Fleming, John Nebel, Mipko, Alicja Kaminska, Slavko Radman, and David Poole.
My special thanks to Embarcadero | ![]() |
My special thanks to PVS-Studio | ![]() |
My special thanks to Tipi.build | ![]() |
My special thanks to Take Up Code | ![]() |
My special thanks to SHAVEDYAKS | ![]() |
Modernes C++ GmbH
Modernes C++ Mentoring (English)
Rainer Grimm
Yalovastraße 20
72108 Rottenburg
Mail: schulung@ModernesCpp.de
Mentoring: www.ModernesCpp.org
Leave a Reply
Want to join the discussion?Feel free to contribute!