C++20: Displaying and Checking Calendar Dates
I created calendar dates in my last post, “C++20: Creating Calendar Dates,” and I will display and check them today.
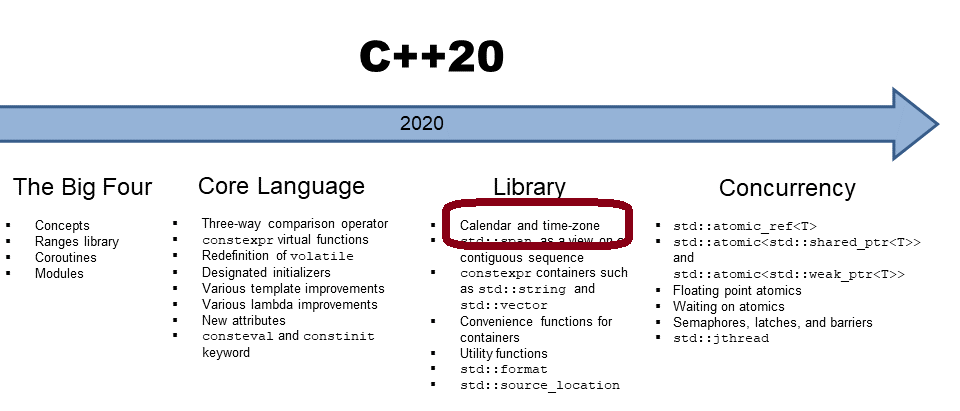
This post is the fifth in my detailed journey through the chrono extension in C++20:
- Basic Chrono Terminology
- Basic Chrono Terminology with Time Duration and Time Point
- Time of Day: Details
- C++20: Creating Calendar Dates
Displaying Calendar Dates
Thanks to std::chrono::local_days
or std::chrono::sys_days
, you can convert calendar dates to a local or a system std::chrono::time_point
. I use std::chrono::sys_days
in my example. std::chrono::sys_days
is based on std::chrono::system_clock
.
// sysDays.cpp #include <chrono> #include <iostream> int main() { std::cout << '\n'; using std::chrono::last; using std::chrono::year; using std::chrono::sys_days; using std::chrono::March; using std::chrono::February; using std::chrono::Monday; using std::chrono::Thursday; constexpr auto yearMonthDayLast{year(2010)/March/last}; // (1) std::cout << "sys_days(yearMonthDayLast): " << sys_days(yearMonthDayLast) << '\n'; constexpr auto yearMonthWeekday{year(2020)/March/Thursday[2]}; std::cout << "sys_days(yearMonthWeekday): " << sys_days(yearMonthWeekday) << '\n'; constexpr auto yearMonthWeekdayLast{year(2010)/March/Monday[last]}; std::cout << "sys_days(yearMonthWeekdayLast): " << sys_days(yearMonthWeekdayLast) << '\n'; std::cout << '\n'; constexpr auto leapDate{year(2012)/February/last}; std::cout << "sys_days(leapDate): " << sys_days(leapDate) << '\n'; // (2) constexpr auto noLeapDate{year(2013)/February/last}; std::cout << "sys_day(noLeapDate): " << sys_days(noLeapDate) << '\n'; // (3) std::cout << '\n'; }
The std::chrono::last
constant (line 1) lets me quickly determine how many days a month has. The output shows that 2012 is a leap year (line 2), but not 2013 (line 3).
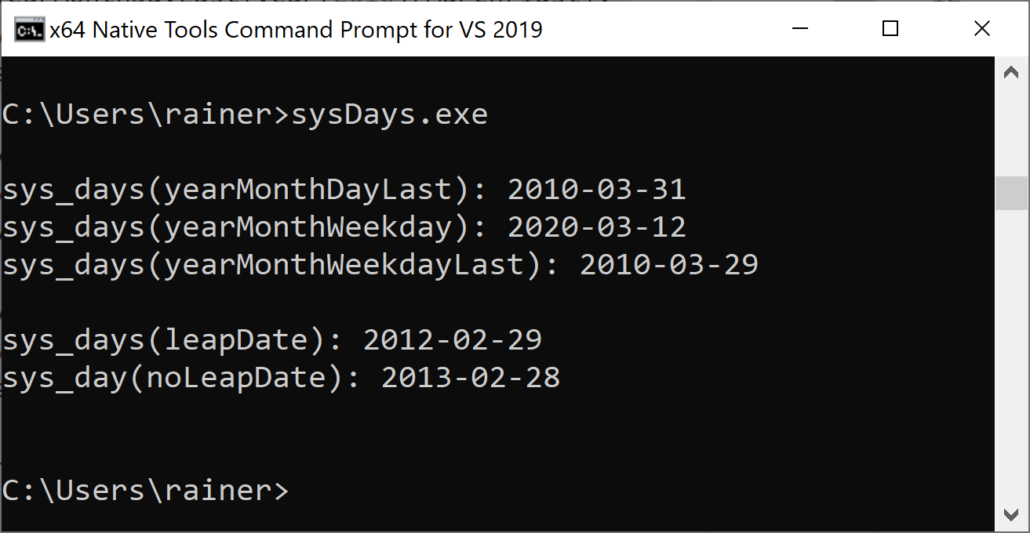
Suppose you have a calendar date like year(2100)/2/29
. Your first question may be: Is this date valid?
Check if a Date is Valid
The various calendar types in C++20 have the function ok
. This function returns true
if the date is valid.
// leapYear.cpp #include <chrono> #include <iostream> int main() { std::cout << std::boolalpha << '\n'; std::cout << "Valid days" << '\n'; // (1) std::chrono::day day31(31); std::chrono::day day32 = day31 + std::chrono::days(1); std::cout << " day31: " << day31 << "; "; std::cout << "day31.ok(): " << day31.ok() << '\n'; std::cout << " day32: " << day32 << "; "; std::cout << "day32.ok(): " << day32.ok() << '\n'; std::cout << '\n'; std::cout << "Valid months" << '\n'; // (2) std::chrono::month month1(1); std::chrono::month month0(0); std::cout << " month1: " << month1 << "; "; std::cout << "month1.ok(): " << month1.ok() << '\n'; std::cout << " month0: " << month0 << "; "; std::cout << "month0.ok(): " << month0.ok() << '\n'; std::cout << '\n'; std::cout << "Valid years" << '\n'; // (3) std::chrono::year year2020(2020); std::chrono::year year32768(-32768); std::cout << " year2020: " << year2020 << "; "; std::cout << "year2020.ok(): " << year2020.ok() << '\n'; std::cout << " year32768: " << year32768 << "; "; std::cout << "year32768.ok(): " << year32768.ok() << '\n'; std::cout << '\n'; std::cout << "Leap Years" << '\n'; constexpr auto leapYear2016{std::chrono::year(2016)/2/29}; constexpr auto leapYear2020{std::chrono::year(2020)/2/29}; constexpr auto leapYear2024{std::chrono::year(2024)/2/29}; std::cout << " leapYear2016.ok(): " << leapYear2016.ok() << '\n'; std::cout << " leapYear2020.ok(): " << leapYear2020.ok() << '\n'; std::cout << " leapYear2024.ok(): " << leapYear2024.ok() << '\n'; std::cout << '\n'; std::cout << "No Leap Years" << '\n'; constexpr auto leapYear2100{std::chrono::year(2100)/2/29}; constexpr auto leapYear2200{std::chrono::year(2200)/2/29}; constexpr auto leapYear2300{std::chrono::year(2300)/2/29}; std::cout << " leapYear2100.ok(): " << leapYear2100.ok() << '\n'; std::cout << " leapYear2200.ok(): " << leapYear2200.ok() << '\n'; std::cout << " leapYear2300.ok(): " << leapYear2300.ok() << '\n'; std::cout << '\n'; std::cout << "Leap Years" << '\n'; constexpr auto leapYear2000{std::chrono::year(2000)/2/29}; constexpr auto leapYear2400{std::chrono::year(2400)/2/29}; constexpr auto leapYear2800{std::chrono::year(2800)/2/29}; std::cout << " leapYear2000.ok(): " << leapYear2000.ok() << '\n'; std::cout << " leapYear2400.ok(): " << leapYear2400.ok() << '\n'; std::cout << " leapYear2800.ok(): " << leapYear2800.ok() << '\n'; std::cout << '\n'; }
I check in the program if a given day (line 1), a given month (line 2), or a given year (line 3) is valid. The range of a day is [1, 31], of a month [1, 12], and a year [ -32767, 32767]. Consequently, the ok()
calls on the corresponding values return false
. Two facts are interesting when I display various values. First, if the value is not valid, the output shows: “is not a valid day”, “is not a valid month”, “is not a valid year”. Second, the values are displayed in string representation.
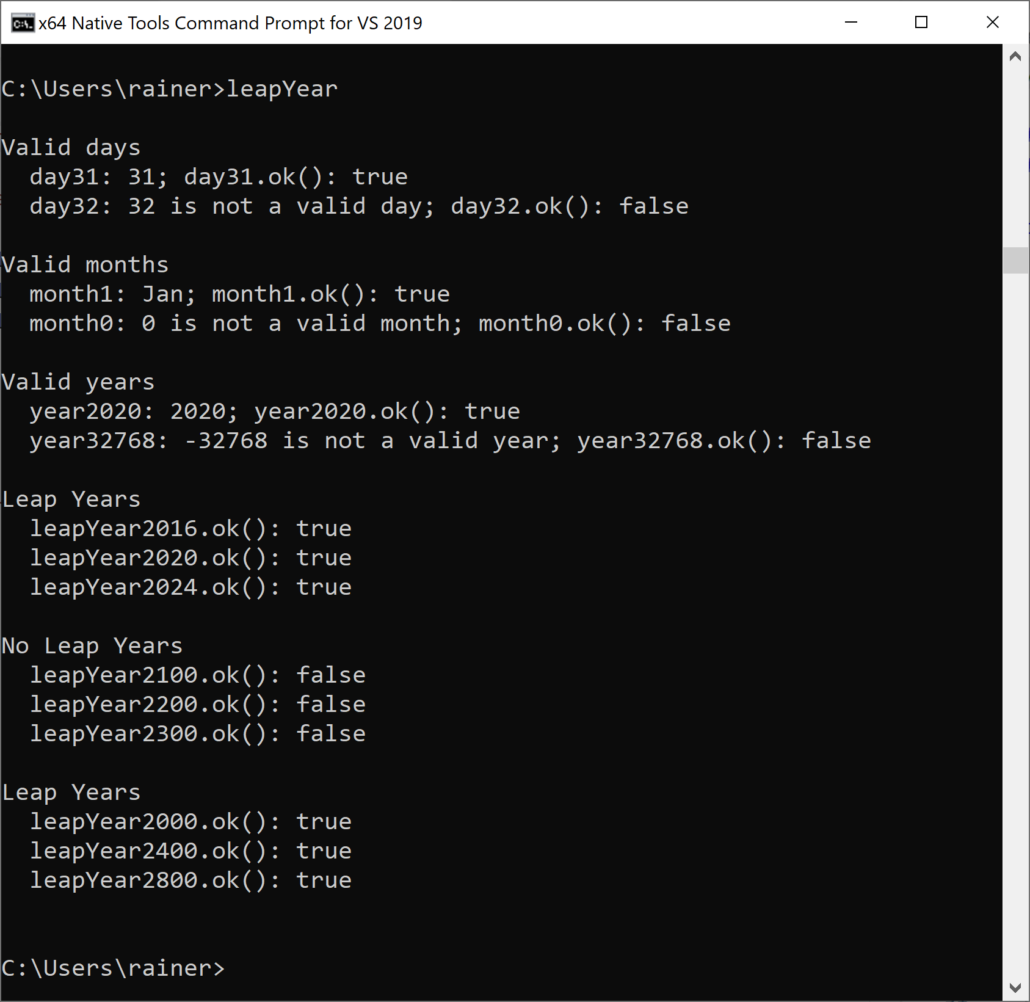
You can apply the ok
-call on a calendar date. Now it’s pretty easy to check if a specific calendar date is a leap day and, therefore, the corresponding year a leap year. In the worldwide used Gregorian calendar, the following rules apply:
Each year that is exactly divisible by 4 is a leap year.
- Except for years that are exactly divisible by 100. They are not leap years.
- Except for years that are exactly divisible by 400. They are leap years.
Too complicated? The program leapYears.cpp
exemplifies this rule.
Modernes C++ Mentoring
Be part of my mentoring programs:
Do you want to stay informed: Subscribe.
What’s Next?
The extended chrono library makes it relatively easy to ask for the time duration between calendar dates.
Thanks a lot to my Patreon Supporters: Matt Braun, Roman Postanciuc, Tobias Zindl, G Prvulovic, Reinhold Dröge, Abernitzke, Frank Grimm, Sakib, Broeserl, António Pina, Sergey Agafyin, Андрей Бурмистров, Jake, GS, Lawton Shoemake, Jozo Leko, John Breland, Venkat Nandam, Jose Francisco, Douglas Tinkham, Kuchlong Kuchlong, Robert Blanch, Truels Wissneth, Kris Kafka, Mario Luoni, Friedrich Huber, lennonli, Pramod Tikare Muralidhara, Peter Ware, Daniel Hufschläger, Alessandro Pezzato, Bob Perry, Satish Vangipuram, Andi Ireland, Richard Ohnemus, Michael Dunsky, Leo Goodstadt, John Wiederhirn, Yacob Cohen-Arazi, Florian Tischler, Robin Furness, Michael Young, Holger Detering, Bernd Mühlhaus, Stephen Kelley, Kyle Dean, Tusar Palauri, Juan Dent, George Liao, Daniel Ceperley, Jon T Hess, Stephen Totten, Wolfgang Fütterer, Matthias Grün, Phillip Diekmann, Ben Atakora, Ann Shatoff, Rob North, Bhavith C Achar, Marco Parri Empoli, moon, Philipp Lenk, Hobsbawm, Charles-Jianye Chen, and Keith Jeffery.
Thanks, in particular, to Jon Hess, Lakshman, Christian Wittenhorst, Sherhy Pyton, Dendi Suhubdy, Sudhakar Belagurusamy, Richard Sargeant, Rusty Fleming, John Nebel, Mipko, Alicja Kaminska, Slavko Radman, and David Poole.
My special thanks to Embarcadero | ![]() |
My special thanks to PVS-Studio | ![]() |
My special thanks to Tipi.build | ![]() |
My special thanks to Take Up Code | ![]() |
My special thanks to SHAVEDYAKS | ![]() |
Seminars
I’m happy to give online seminars or face-to-face seminars worldwide. Please call me if you have any questions.
Standard Seminars (English/German)
Here is a compilation of my standard seminars. These seminars are only meant to give you a first orientation.
- C++ – The Core Language
- C++ – The Standard Library
- C++ – Compact
- C++11 and C++14
- Concurrency with Modern C++
- Design Pattern and Architectural Pattern with C++
- Embedded Programming with Modern C++
- Generic Programming (Templates) with C++
- Clean Code with Modern C++
- C++20
Online Seminars (German)
- Clean Code: Best Practices für modernes C++ (21. Mai 2024 bis 23. Mai 2024)
- Embedded
Programmierung mit modernem C++ (2. Jul 2024 bis 4.
Jul 2024)
Contact Me
- Phone: +49 7472 917441
- Mobil:: +49 176 5506 5086
- Mail: schulung@ModernesCpp.de
- German Seminar Page: www.ModernesCpp.de
- Mentoring Page: www.ModernesCpp.org
Modernes C++ Mentoring,